Hello Everyone, This is me Nonthakon again. I think that i’m pretty have a plenty of free-time in this weekend. So I decided to making some kind of Chatbot Program. (With Python of Course)
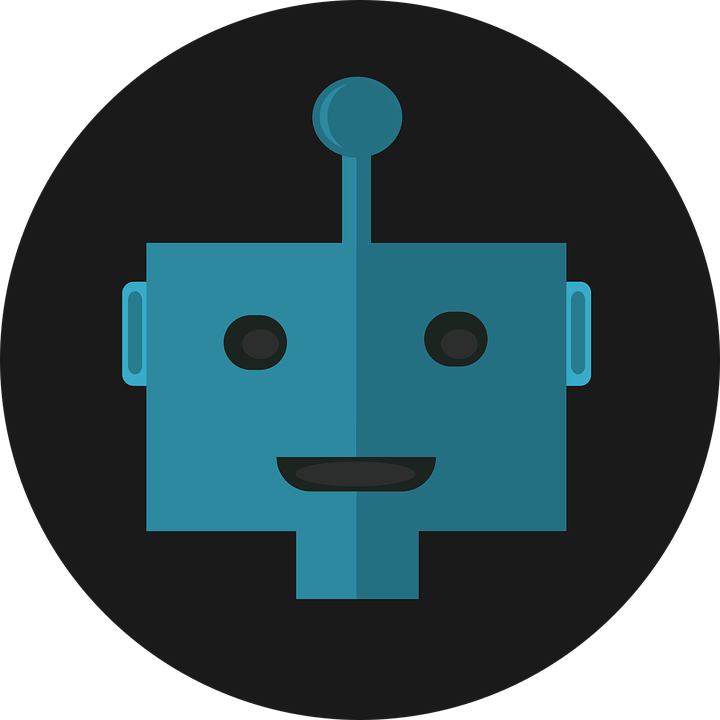
Let’s Start by planning our bot. I want he/she to answer us something like.
- When we say hello he/she could say hello back to us.
- Also with Good bye.
- I might want to ask him/her about time.
Yes, This is good plan!
So What is the module we gonna use?
- datetime for telling us the time
- random because you might don’t want your bot to say only one sentence
- And lastly IntentParser
Damn Cool!
But what is IntentParser? I am not kinda know it? It’s fine if you don’t know it. Because I just wrote it about a week ago. But you might want to check it out!
https://github.com/nonkung51/IntentParser
what it could do is parsing sentence to a computer readable format (dict in python)
e.g. What’s the weather like in California?
=> {'type': 'WeatherIntent', 'confidence': 0.8571428571428571, 'args': [('location', 'California')}
Just check it out!
Anyway Let’s start what we really need to do …Chatbot!
We start by import what we wanna useimport intentparser
import datetime
import random
then let’s make an intentparser for checking the sentence we would recievesallIntent = []HelloIntent = ip.intentParser({
'description' : {
"type" : 'HelloIntent',
"args" : [],
"keyword" : [
(ip.REQUIRE, "hello_keyword"),
]},
'hello_keyword' : ['hello', 'hi'],
})
HelloIntent.teachWords(["Hello, How are you?", "Hi, Mr.John", "Hello, nice to meet you!"])
allIntent.append(HelloIntent)ByeIntent = ip.intentParser({
'description' : {
"type" : 'ByeIntent',
"args" : [],
"keyword" : [
(ip.REQUIRE, "bye_keyword"),
]},
'bye_keyword' : ['bye'],
})
ByeIntent.teachWords(["Good bye.", "Bye, Josh.", "Good bye, Be safe."])
allIntent.append(ByeIntent)TimeIntent = ip.intentParser({
'description' : {
"type" : 'TimeIntent',
"args" : [(ip.OPTIONAL, "scopes")],
"keyword" : [
(ip.REQUIRE, "time_keyword"),
(ip.OPTIONAL, "scopes")
]},
'time_keyword' : ['is', 'are', 'what', 'how', 'clock', 'how\'s'],
'scopes' : [
"day",
"time"
]
})
TimeIntent.teachWords(["What time is it?", "How's the clock", "What is this day"])
allIntent.append(TimeIntent)
as you can see that we append our intent to allIntent. But why?
since we didn’t have only one intent. that make us confuse what do we actually need to answer for.
imagine if you have a chance to answer question in 3 ways.
- say hello
- say bye
- or tell the time
To only one question. You might confuse what is the answer you need to say in that situation? like if i say “Hello”. Would it be good if you tell me the time?
It’s not. That’s why we have something call confidence point. It’s use to recognize what you really talking about. like if I say “Hello” again.
The HelloIntent might say that “Oh my confidence is 0.9”. But ByeIntent might have only 0.4 or something. And maybe TimeIntent might have 0
This is easier now you know. What you really want to answer.
We making a list allIntent to make it easier to compare confidence in every intent that we use (using max()).
Next, we making a loop for every sentence we say to our bot.while True:
Then, we get an input. And set new variable to store temporary variable.text = input('User said : ').lower()
temp = []
Next,for i in allIntent:
_temp = i.getResult(text)
try:
temp.append((_temp['confidence'], _temp['type']))
except Exception as e:
pass
For every element in allIntent we gonna append tuples of confidence and type of intent into our temporary variable.try:
candidate = max(temp)
if candidate[1] == 'HelloIntent':
print(random.choice(['Chatbot said : Hello!',
'Chatbot said : How are you?',
'Chatbot said : What\'s up!']))
try to defining our candidate by using max() to find the highest confidence intent. If our candidate[1] (type of intent) is ‘HelloIntent’. We gonna answer something in the list(this is why we need random because you might don’t want them to answer only one thing all the time.)elif candidate[1] == 'ByeIntent':
print(random.choice(['Chatbot said : Good bye!',
'Chatbot said : Good Luck!',
'Chatbot said : See ya!']))
break
This is the same as above. But after we said bye we would break. And the Program would stop.elif candidate[1] == 'TimeIntent':
typeOfTime = TimeIntent.getResult(text)['args']
typeOfTime = [item for item in typeOfTime if item[0] == 'scopes'][0][1]
now = datetime.datetime.now()
if typeOfTime == ['day']:
print("Chatbot said : Today is {}/{}/{}".format(now.day, now.month, now.year))
else:
print("Chatbot said : It's {}:{}".format(now.hour, now.minute if now.minute > 9 else "0" + str(now.minute)))
del typeOfTime, now
This is the case when we want to know the time. We gonna check the type of TimeIntent first(day or time) then we gonna answer and clean used variable after that.else:
print('error')
del text, temp, _temp, candidate
except Exception as e:
print('Error')
if we can’t find any intent we would say ‘error’. and if we encounter any error we would say the same.
That’s all! Let’s test!
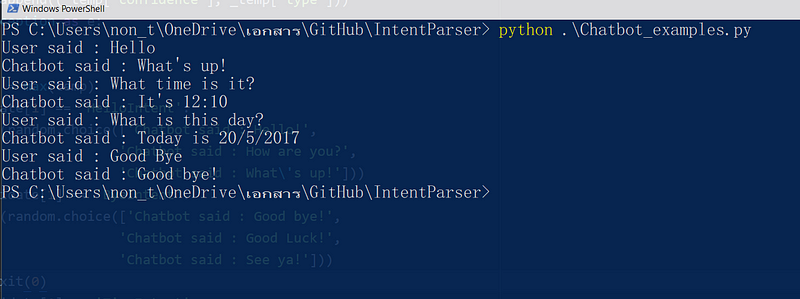
This is all of it. So thank you for reading this anyway. See ya next time!
Don’t forget to check this out! :
- https://goo.gl/qRcw1e // the intentparser
- https://goo.gl/agwF7A //Making program that warn you to rest your eyes (with python)